Bookmarklets – Powerful and almost forgotten
What the hell is a bookmarklet? If you are not familiar with it and want to know more about it, then this is for you. We are going to go through the technical basics, why there are so useful and how to create one.
In a nutshell
As the name indicates, or you might have already guessed, it has something to do with bookmarks. When you click on them, normally the page you saved gets opened. But that's boring!
What if I told you JavaScript is also a valid URL? In the early days of the internet, when it was introduced, it was very common for websites to use it that way. Also for dummy links you might see javascript:void(0), nowadays, this is no longer up to date and a good idea.
So, what happens in this case is: nothing. Yes, you are reading right. It was used for example to make links clickable that did nothing (to listen on the event). You can basically call any function this way, also anonymous, aka inline functions.
This means the world of JavaScript is yours. Okay, there are limitations to be fair. But you will probably never reach them anyway.
Back in the days
A few years ago, this was a common thing for example to use in link shorteners. bit.ly was one of the most prominent examples of this. However, it was used like basically all over the place.
Neat use cases everywhere
Let's say you have an external tool/website you want to extract data from. Or modify the page to add some custom stuff to make your life easier. As long as you can do it with JavaScript, it's possible.
How to build one
So, you might think, bookmarklets are nice. But they seem complicated to make? No, not all!
Let's get started.
The base
The base is an anonymous function we define and immediately call.
(function() {
})()
Add some spice
Let's add an alert when you click the bookmarklet.
(function() {
alert("Hello, from the bookmarklet!")
})()
Make it executable
Now, we have the function ready. However, we will need to make it URL-compatible.
This means:
- Making it a single line of code
- URL-encode it
The simplest solution is to use the JavaScript console, or if you are lazy the good old urlencoder.org page:
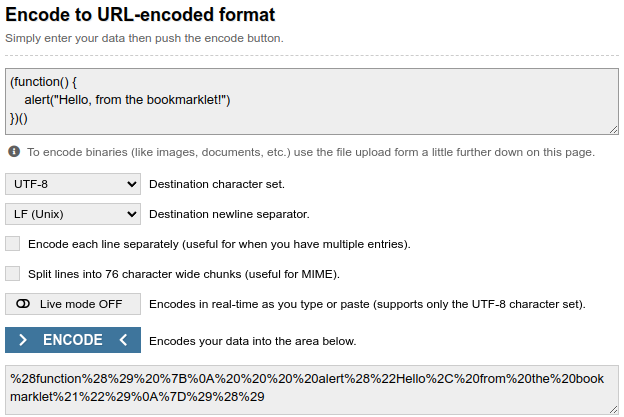
So, we have the code part, let's prepend javascript: and we have a working bookmarklet.
javascript:%28function%28%29%20%7B%0A%20%20%20%20alert%28%22Hello%2C%20from%20the%20bookmarklet%21%22%29%0A%7D%29%28%29
Now create a new bookmark with this text as the URL. When you click it, you should see something like this:
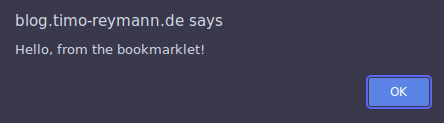
Development
When you are developing bookmarklets, chances are that you are not already clear about what you need. That's fine, and the best way to get started is via the browser console. It is mighty and allows you to create variables, functions etc.
After all, it's an interactive JavaScript interpreter. Meaning, you can iteratively develop it and then glue everything together in the bookmarklet.
Made up example: Get all secondary headings
Let's say you want to get all secondary headings of a webpage, for whatever reason, and get the count in an alert.
Get the headings with the querySelectorAll. First set the variable containing all the text contents. Checking is as simple as writing the variable name, printing the contents in a nicely formatted manner:

And now we want to get these count as an alert, so just call alert and watch the magic happen:

Now combine everything together:
(function() {
const headingsLevel2 = Array.from(document.querySelectorAll("h2")).map(heading => heading.innerText)
alert(`Found ${headingsLevel2.length} subheadings`)
})()
Urlencode and prepend javascript: and it's ready to use:
javascript:%28function%28%29%20%7B%0A%20%20%20%20const%20headingsLevel2%20%3D%20Array.from%28document.querySelectorAll%28%22h2%22%29%29.map%28heading%20%3D%3E%20heading.innerText%29%0A%20%20%20%20alert%28%60Found%20%24%7BheadingsLevel2.length%7D%20subheadings%60%29%0A%7D%29%28%29
Save it as a bookmark and voilà , it's done! :)
Conclusion
Bookmarklets are still powerful and there are a lot of use cases and things where they are helpful. They are easy to create and the steps shown are easily automatable.
Plenty of generators, ready to use, are already available out there. Just use Google or even create your own, if you'd like to. Otherwise, you can also use my bookmarklet generator: bookmarklet-generator.timo-reymann.de.